Dailies: Taking an hour each day to prototype and learn new techniques.
Collection of tutorials and assets from Pluralsight
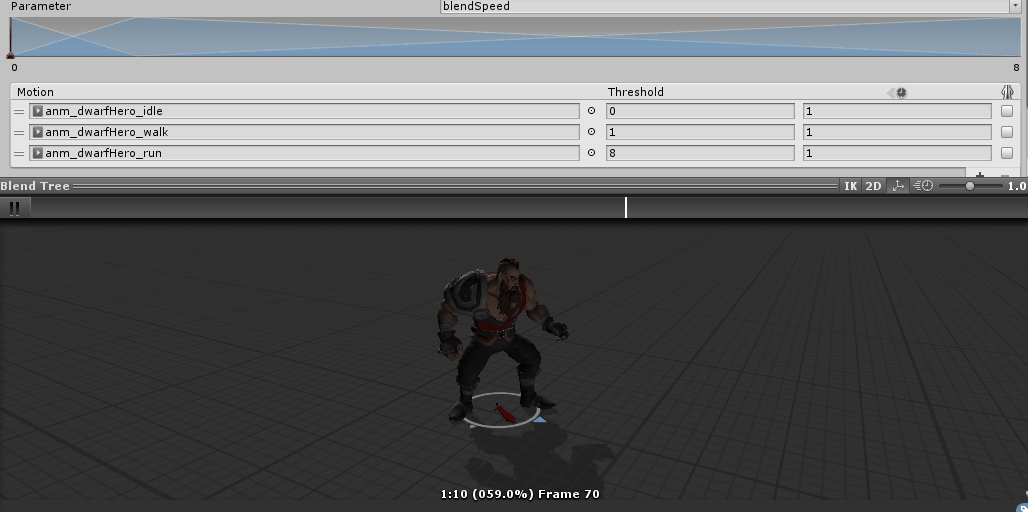
Digging into gameplay programming, how to build everything I now know together to get some working gameplay.
Starting off setting up the animator to the player
Daily 18.11.20 /// 165 // animator / #madewithunity #gamedev
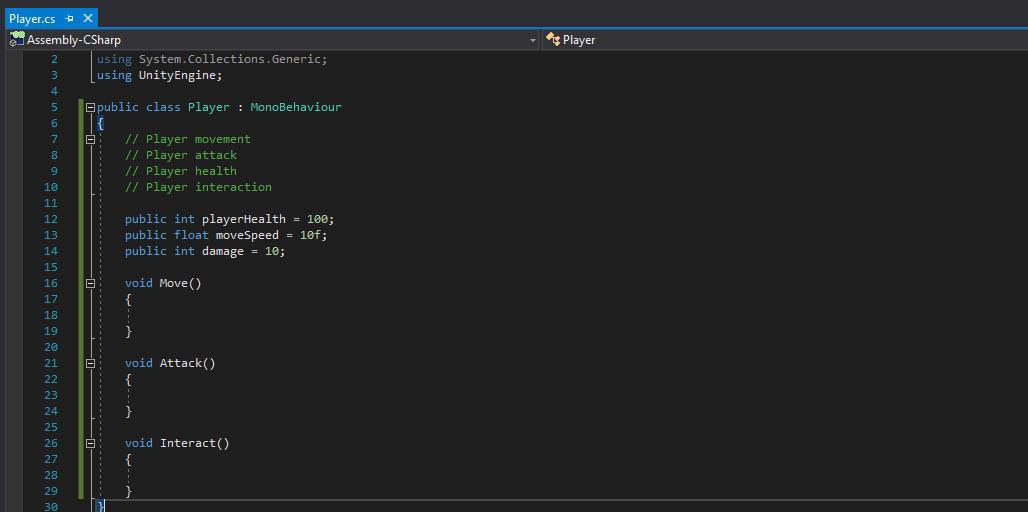
Digging into gameplay programming, how to build everything I now know together to get some working gameplay.
Learning about pseudocode and how powerful it can be to help breakdown your C# scripts.
noobandIknowit
Daily 18.11.20 /// 165 // pseudocode / #madewithunity #gamedev
Well here we go, it’s time to start scripting some C#. I’m excited about this one, I’ve dabbled with code many times but never to the depth of C#. Time to role up the sleeves and embrace the code.
This is a course from Pluralsight focusing on scripting within the Unity game engine.
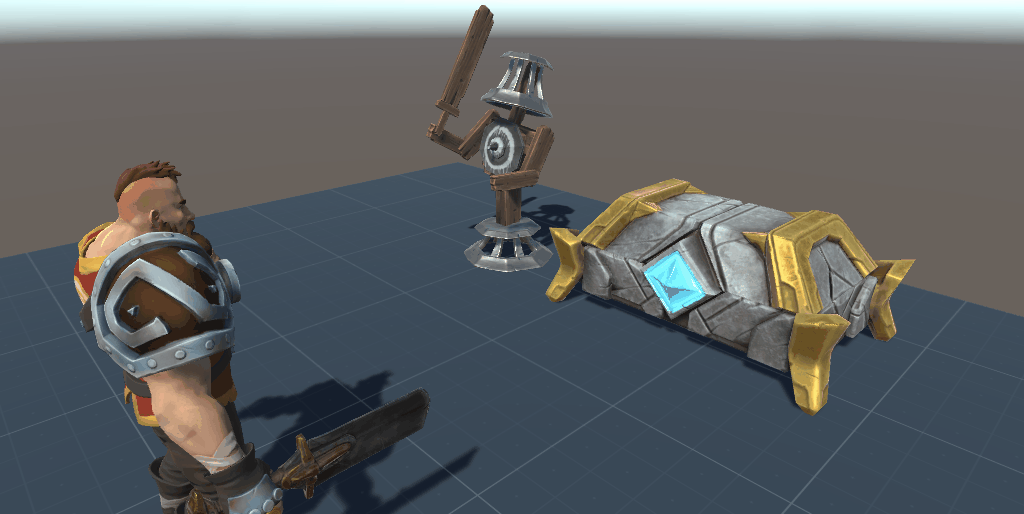
Finishing up my C# fundamentals dailies & really enjoyed it.
Not much to look at here but I’ve learned script communication, using collisions, triggers, raycasting, instantiation & destruction.
Powerful stuff
Daily 18.10.02 /// 139 // object interaction / #madewithunity #gamedev
TreasureChest.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TreasureChest : MonoBehaviour
{
public bool interactable = false;
private Animator anim;
public Rigidbody coinPrefab;
public Transform spawner;
// Use this for initialization
void Start ()
{
anim = GetComponent<Animator>();
}
// Update is called once per frame
void Update ()
{
if(interactable == true && Input.GetKeyDown(KeyCode.Space))
{
anim.SetBool("openChest", true);
Rigidbody coinInstance;
coinInstance = Instantiate(coinPrefab, spawner.position, spawner.rotation) as Rigidbody;
coinInstance.AddForce(spawner.up * 100);
}
}
void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
interactable = true;
}
}
private void OnTriggerExit(Collider other)
{
if(other.gameObject.tag =="Player")
{
interactable = false;
}
}
}
Player.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour {
public CapsuleCollider playerCollider;
public float moveSpeed = 5f;
private Enemy enemyScript;
private RaycastHit hit;
private Ray ray;
public float rayDistance = 4f;
// Use this for initialization
void Start ()
{
playerCollider = GetComponent<CapsuleCollider>();
playerCollider.height = 1f;
playerCollider.center = new Vector3(0f, 0.5f, 0f);
}
// Update is called once per frame
void Update ()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0f, moveVertical);
transform.Translate(movement * Time.deltaTime * moveSpeed);
ray = new Ray(transform.position + new Vector3(0f,playerCollider.center.y, 0f), transform.forward);
Debug.DrawRay(ray.origin, ray.direction * rayDistance, Color.red);
if(Physics.Raycast(ray, out hit))
{
if(hit.distance < rayDistance)
{
if (hit.collider.gameObject.tag == "Enemy")
print("There is an enemy ahead");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.tag == "Enemy")
{
enemyScript = collision.gameObject.GetComponent<Enemy>();
enemyScript.enemyHealth--;
}
}
}
Coin.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Coin : MonoBehaviour
{
// Use this for initialization
void Start ()
{
Destroy(gameObject, 2.0f);
}
// Update is called once per frame
void Update ()
{
}
}
Enemy.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Enemy : MonoBehaviour
{
public int enemyHealth = 4;
// Use this for initialization
void Start ()
{
}
// Update is called once per frame
void Update ()
{
}
}
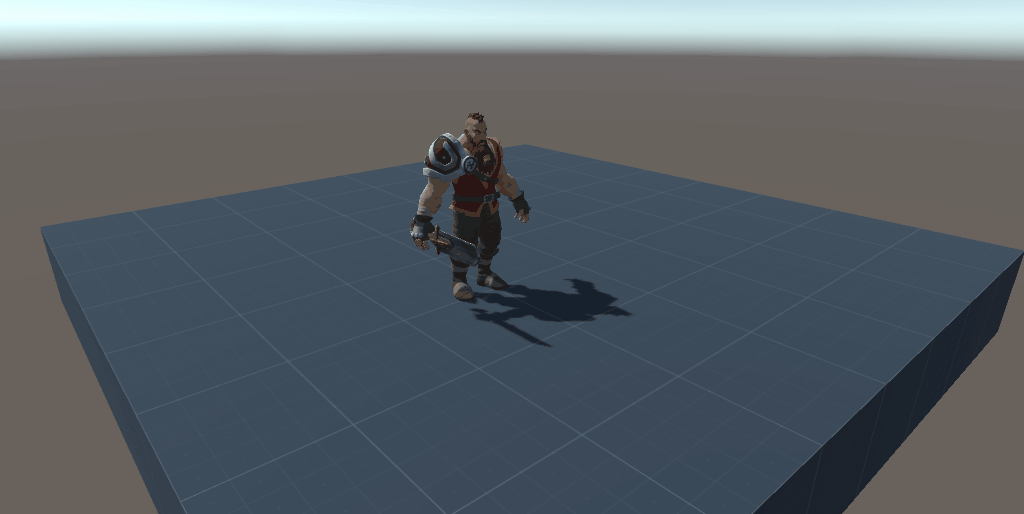
Getting an understanding of how to access an objects components and manipulate them
Daily 18.10.01 /// 138 // object manipulation / #unity #madewithunity #gamedev
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour {
public CapsuleCollider playerCollider;
public float moveSpeed = 5f;
// Use this for initialization
void Start ()
{
playerCollider = GetComponent<CapsuleCollider>();
playerCollider.height = 1f;
playerCollider.center = new Vector3(0f, 0.5f, 0f);
}
// Update is called once per frame
void Update ()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0f, moveVertical);
transform.Translate(movement * Time.deltaTime * moveSpeed);
}
}
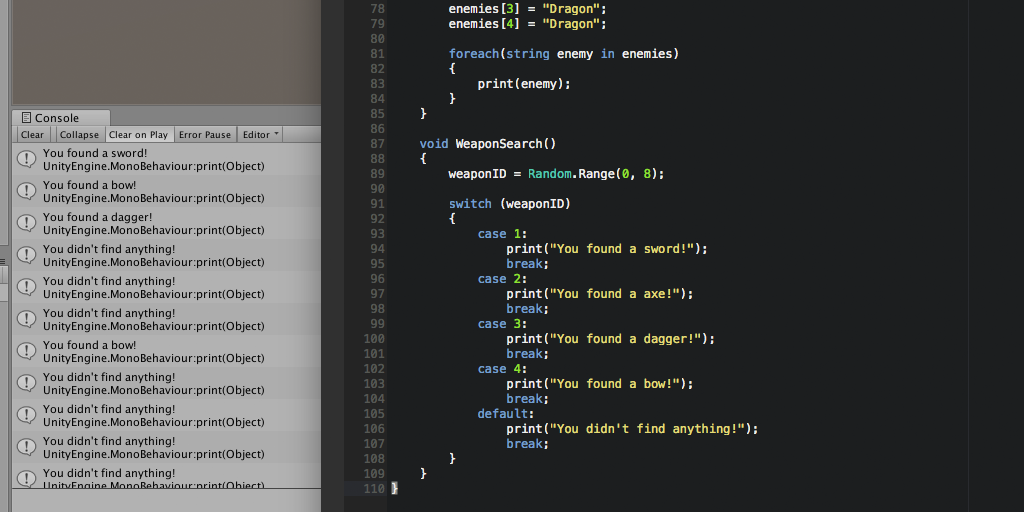
Continuing on my 1hr daily to learn C#.
Today learning all the if, for, while, do and switch statements.
Massive respect to #gamedevs who can do so much with so little, hope to be in that club soon
Daily 18.09.28 /// 137 // code flow / #unity #madewithunity #gamedev
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ExampleScript_B : MonoBehaviour
{
private int enemyDistance = 0;
private int enemyCount = 10;
private string[] enemies = new string[5];
private int weaponID = 0;
// Use this for initialization
void Start ()
{
}
// Update is called once per frame
void Update ()
{
if(Input.GetKeyUp("space"))
{
//EnemySearch();
//EnemyDestruction();
//EnemyScan();
//EnemyRoster();
WeaponSearch();
}
}
void EnemySearch()
{
for (int i = 0; i < 5; i++)
{
enemyDistance = Random.Range(1, 10);
if (enemyDistance >= 8)
{
print("Enemy is far away!");
}
if (enemyDistance >= 4 && enemyDistance <= 7)
{
print("Enemy is at medium range");
}
if (enemyDistance < 4)
{
print("Enemy is very close!");
}
}
}
void EnemyDestruction()
{
while(enemyCount > 0)
{
print("There is an enemy! Let's destroy it!");
enemyCount--;
}
}
void EnemyScan()
{
bool isAlive = false;
do
{
print("Scanning for enemies!");
}
while (isAlive == true);
}
void EnemyRoster()
{
enemies[0] = "Ork";
enemies[1] = "Dragon";
enemies[2] = "Ork";
enemies[3] = "Dragon";
enemies[4] = "Dragon";
foreach(string enemy in enemies)
{
print(enemy);
}
}
void WeaponSearch()
{
weaponID = Random.Range(0, 8);
switch (weaponID)
{
case 1:
print("You found a sword!");
break;
case 2:
print("You found a axe!");
break;
case 3:
print("You found a dagger!");
break;
case 4:
print("You found a bow!");
break;
default:
print("You didn't find anything!");
break;
}
}
}
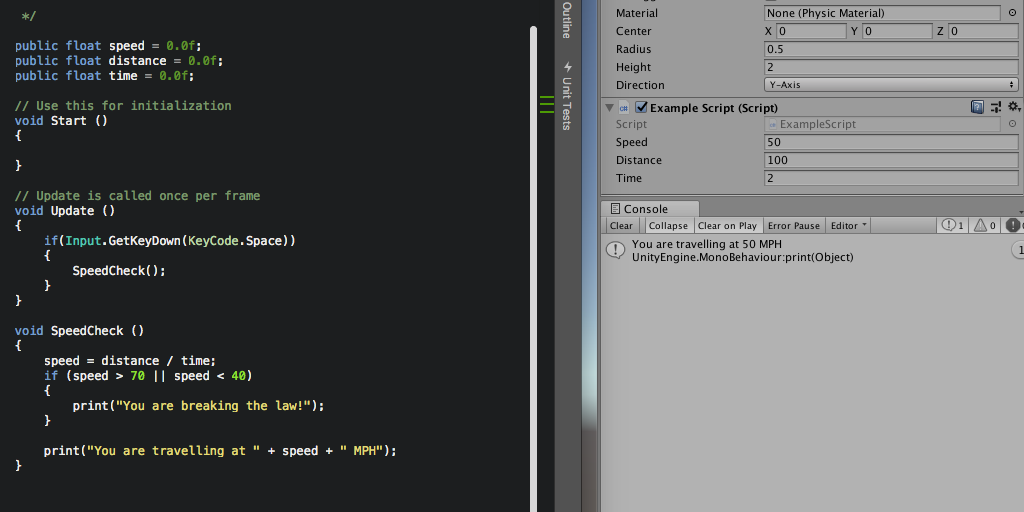
Learning C# in @unity3d, nice and easy introduction and nice of @PolyconArtist not to scare the bejesus out of me straight away.
Excited to dig in deeper.
Daily 18.09.26 /// 135 // c# basics / #unity #madewithunity #gamedev #pluralsight
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ExampleScript : MonoBehaviour {
/*
Assignment Operators:
=
+= speed = speed + y (speed+=y)
-=
*=
/=
%= 7 % 3 = 1
Arithmetic Operators
+
-
*
/
%
Comparison Operators
== Is equal to
!= Not equal to
>
<
>=
<=
Logical Operators
&& And
|| Or
! Not
*/
public float speed = 0.0f;
public float distance = 0.0f;
public float time = 0.0f;
public float maxSpeedLimit = 70f;
public float minSpeedLimit = 40f;
// Use this for initialization
void Start ()
{
}
// Update is called once per frame
void Update ()
{
if(Input.GetKeyDown(KeyCode.Space))
{
SpeedCheck();
}
}
void SpeedCheck ()
{
speed = distance / time;
if(speed > maxSpeedLimit)
{
print("You are exceeding the speed limit!");
}
else if(speed < minSpeedLimit)
{
print("You are not going fast enough!");
}
else if(speed == maxSpeedLimit || speed == minSpeedLimit)
{
print("You are very close the breaking the law!");
}
else
{
print("You are within the speed limit");
}
}
}