Dailies: Take some time to learn Processing, a tool that allows you to create art using Java. Loving shaders and creating via nodes, getting into the code has always been a goal. Here I’ll ideate and prototype as I’m learning the Processing tool and Java more in depth. Now focusing on noise and flow fields.
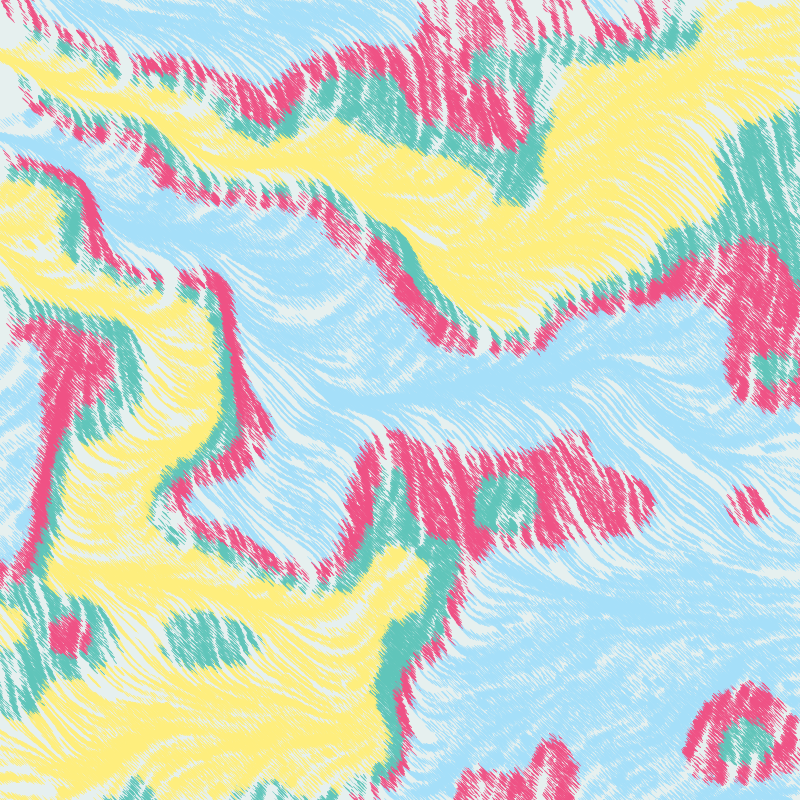
Daily 22.09.22 /// 464 // brush / #CreativeCoding
import java.util.Calendar;
import processing.pdf.*;
Agent[] agents = new Agent[4000];
color[] palette = {#a5dff9, #ef5285, #60c5ba, #feee7d};
int len = 35;
int noiseScale = 500;
int noiseStrength = 20;
long seed = 10000;
long randomSeed;
void setup() {
size(800, 800);
background(#E6F0EF);
noStroke();
noFill();
strokeWeight(3);
randomSeed = (long)random(seed);
println(randomSeed);
noiseSeed(randomSeed);
randomSeed(randomSeed);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (int i = 0; i < agents.length; i++) {
agents[i].display();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed;
color col;
Agent() {
x = random(width);
y = random(height);
speed = random(-5, 5);
col = palette[(int)random(palette.length)];
}
void display() {
for (int i = 0; i < len; i++) {
update();
}
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += sin(angle) * speed;
y += cos(angle) * speed;
int index = int(map(sin(angle), -1, 1, 0, palette.length));
index = constrain(index, 0, palette.length-1);
col = palette[index];
stroke(col);
brush(x, y, (int)random(1, 5));
}
void brush(float posx, float posy, int count) {
pushMatrix();
translate(posx, posy);
for (int i = 0; i < count; i++) {
strokeWeight(random(.25, 1));
float start = random(1, 5);
float end = random(5, 10);
line(start, start, end, end);
}
popMatrix();
}
}
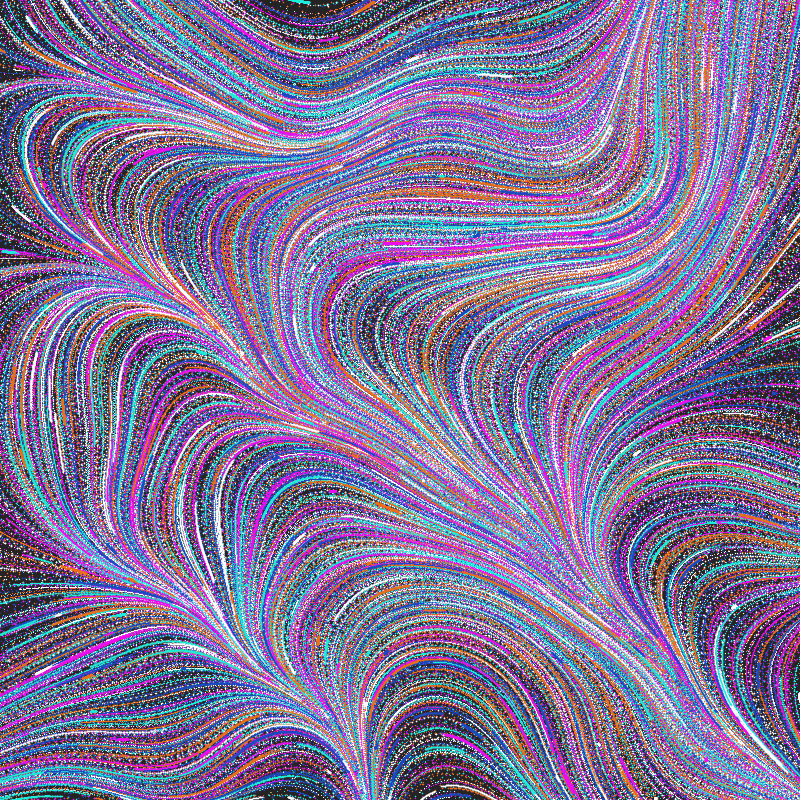
Daily 22.09.20 /// 463 // blinding lights / #CreativeCoding
color[] cols = {#1849C5, #EF0BEF, #EA6F15, #1AF0DC, #FFFFFF}; //Glitch
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[20000];
noiseSeed(7);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
//saveFrame("frames/462-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 1000, noiseStrength = 20;
Agent() {
x = random(width);
y = random(height);
size = random(1, 2);
speed = random(-20, 20);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
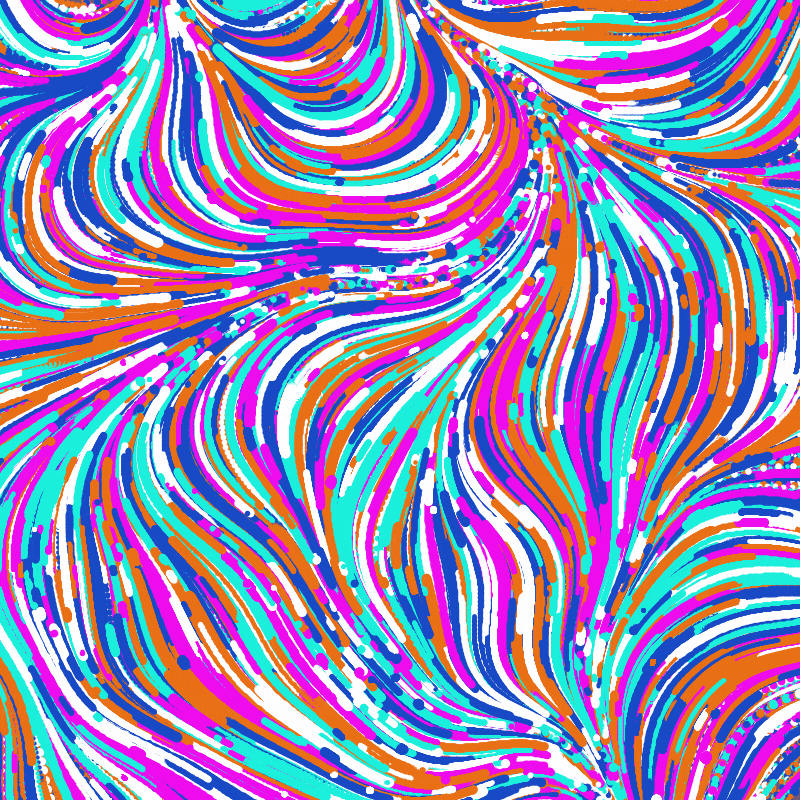
Daily 22.09.18 /// 462 // glitch wave / #CreativeCoding
color[] cols = {#1849C5, #EF0BEF, #EA6F15, #1AF0DC, #FFFFFF}; //Glitch
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[20000];
noiseSeed(5);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/462-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 1000, noiseStrength = 20;
Agent() {
x = random(width);
y = random(height);
size = random(4, 10);
speed = random(-20, 20);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
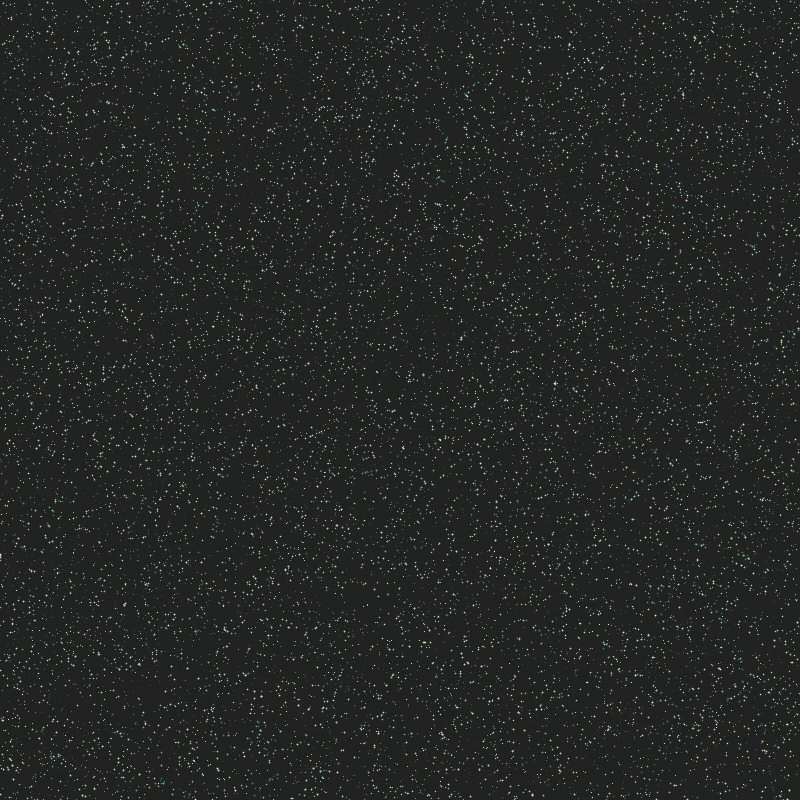
Daily 22.09.16 /// 461 // noise wave / #GenerativeArt
color[] cols = {#cff09e, #79bd9a, #3b8686, #a8dba8};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[20000];
noiseSeed(3);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/461-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 1000, noiseStrength = 10;
Agent() {
x = random(width);
y = random(height);
size = random(0.2, 2);
speed = random(-10, 10);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
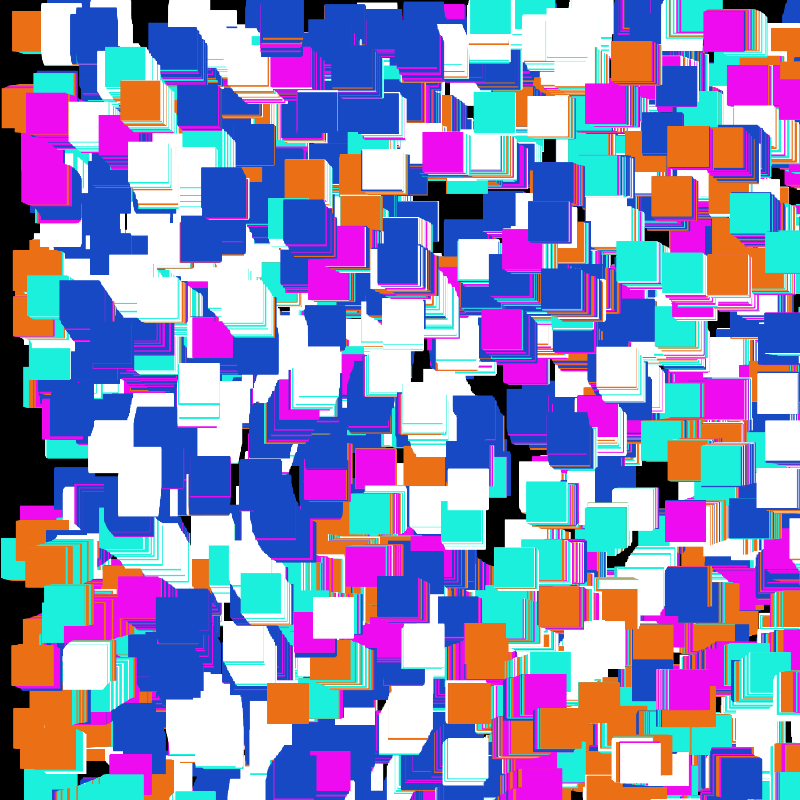
Daily 22.09.15 /// 460 // rect glitch / #GenerativeArt
//color[] palette = {#cff09e, #79bd9a, #3b8686, #a8dba8}; //Greens
//color[] palette = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2}; //Multi
//color[] palette = {#a3daff, #1ec0ff, #0080ff, #03a6ff}; //Blues
color[] palette = {#1849C5, #EF0BEF, #EA6F15, #1AF0DC, #FFFFFF}; //Glitch
Agent[] agents;
void setup() {
size(800, 800);
background(#000000);
agents = new Agent[1000];
noiseSeed(1);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/549b.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 1000, noiseStrength = 1000;
int len = 20;
Agent() {
x = random(width);
y = random(height);
speed = random(-2, 2);
//col = palette[(int)random(palette.length)];
}
void display() {
noStroke();
for (int i = 0; i < len; i++) {
update();
}
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
int index = int(map(sin(angle), -1, 1, 0, palette.length));
index = constrain(index, 0, palette.length-1);
col = palette[index];
fill(col);
brush(x, y, (int)random(1, 4));
}
void brush(float posx, float posy, int count) {
pushMatrix();
translate(posx, posy);
for (int i = 0; i < count; i++) {
rect(20, 10, 40, 40);
}
popMatrix();
}
}
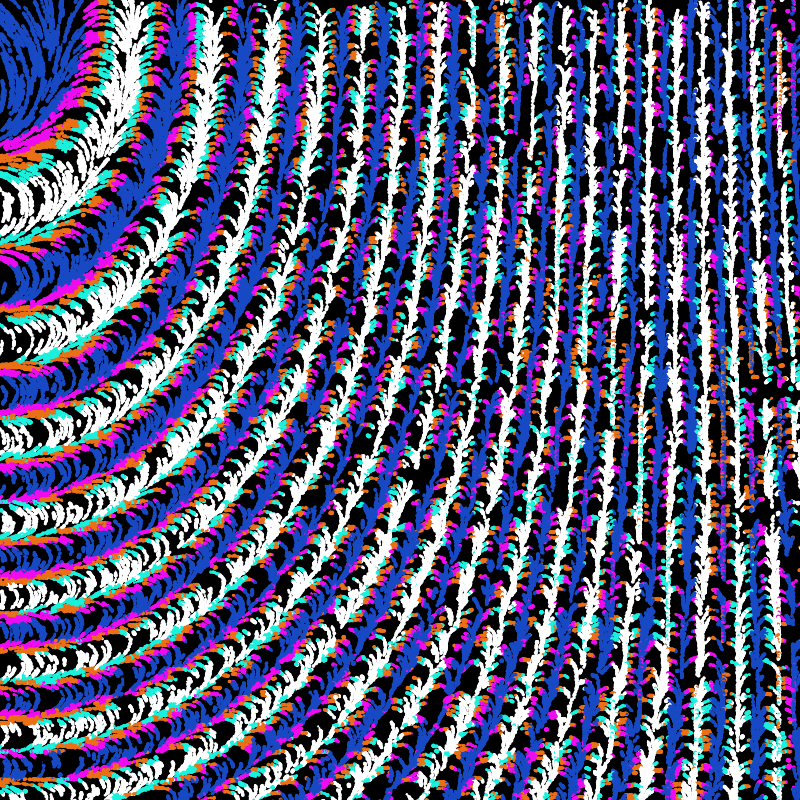
Daily 22.09.13 /// 459 // glitch / #GenerativeArt
//color[] palette = {#cff09e, #79bd9a, #3b8686, #a8dba8}; //Greens
//color[] palette = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2}; //Multi
//color[] palette = {#a3daff, #1ec0ff, #0080ff, #03a6ff}; //Blues
color[] palette = {#1849C5, #EF0BEF, #EA6F15, #1AF0DC, #FFFFFF}; //Glitch
Agent[] agents;
void setup() {
size(800, 800);
background(#000000);
agents = new Agent[10000];
//noiseSeed(5);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/549b.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 10000, noiseStrength = 2000;
int len = 20;
Agent() {
x = random(width);
y = random(height);
speed = random(-2, 2);
//col = palette[(int)random(palette.length)];
}
void display() {
noStroke();
for (int i = 0; i < len; i++) {
update();
}
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
int index = int(map(sin(angle), -1, 1, 0, palette.length));
index = constrain(index, 0, palette.length-1);
col = palette[index];
fill(col);
brush(x, y, (int)random(1, 5));
}
void brush(float posx, float posy, int count) {
pushMatrix();
translate(posx, posy);
for (int i = 0; i < count; i++) {
circle(random(1, 2), random(1, 2), random(1, 4));
}
popMatrix();
}
}
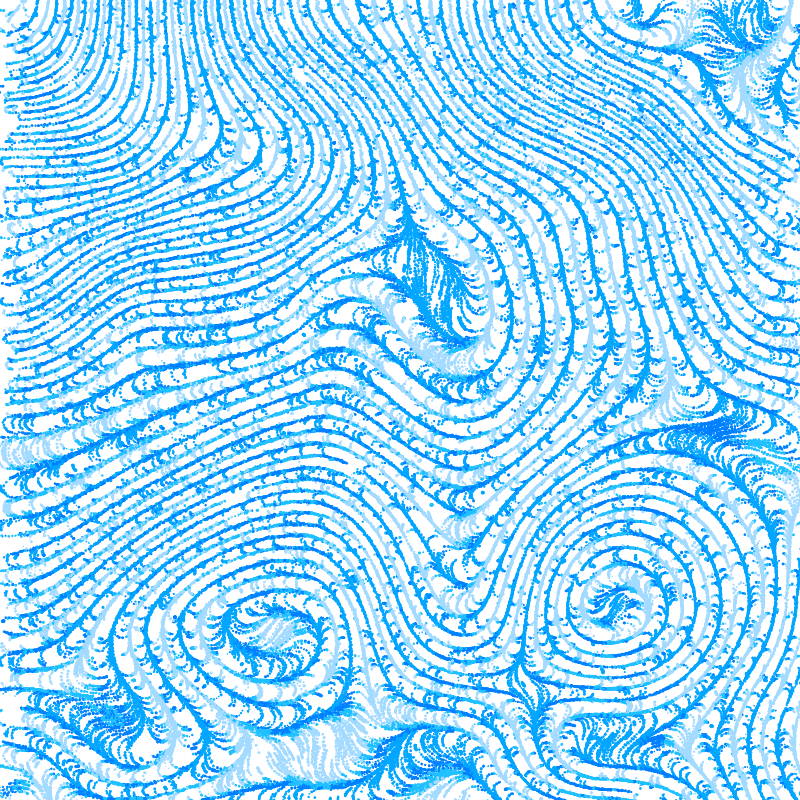
Daily 22.09.12 /// 458 // flow / #GenerativeArt
//color[] palette = {#cff09e, #79bd9a, #3b8686, #a8dba8}; //Greens
//color[] palette = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2}; //Multi
color[] palette = {#a3daff, #1ec0ff, #0080ff, #03a6ff}; //Blues
Agent[] agents;
void setup() {
size(800, 800);
background(#ffffff);
agents = new Agent[10000];
noiseSeed(5);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 1200, noiseStrength = 560;
int len = 20;
Agent() {
x = random(width);
y = random(height);
speed = random(-4, 4);
//col = palette[(int)random(palette.length)];
}
void display() {
noStroke();
for (int i = 0; i < len; i++) {
update();
}
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
int index = int(map(sin(angle), -1, 1, 0, palette.length));
index = constrain(index, 0, palette.length-1);
col = palette[index];
fill(col);
brush(x, y, (int)random(1, 5));
}
void brush(float posx, float posy, int count) {
pushMatrix();
translate(posx, posy);
for (int i = 0; i < count; i++) {
circle(random(1, 2), random(1, 2), random(1, 3));
}
popMatrix();
}
}
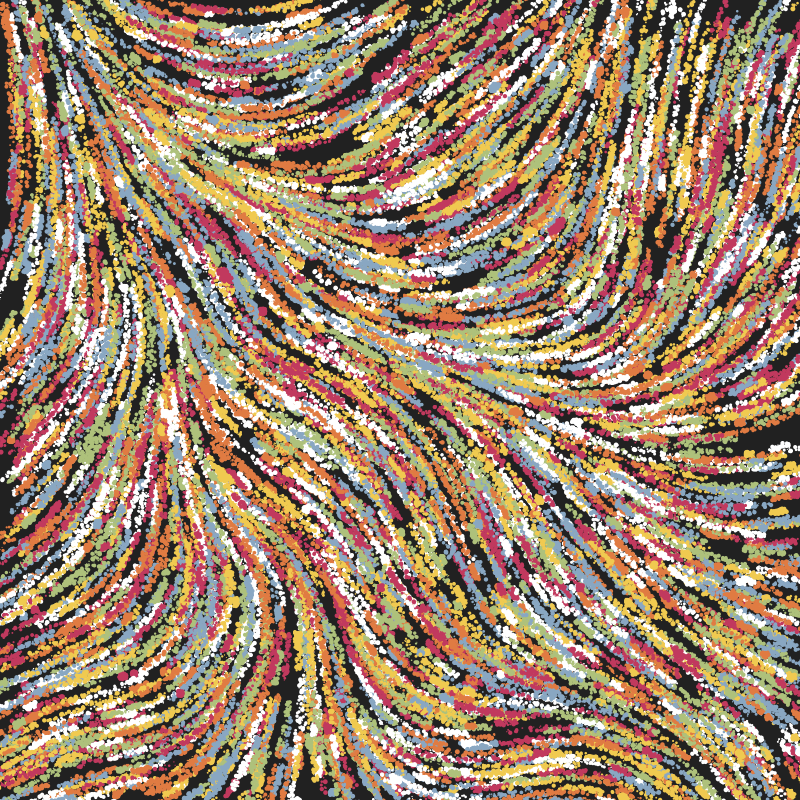
Daily 22.09.09 /// 457 // custom brush / #generativeart #techart #codeart #processing #java
//color[] cols = {#cff09e, #79bd9a, #3b8686, #a8dba8};
color[] cols = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2, #FFFFFF};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[4000];
//noiseSeed(0);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 1000, noiseStrength = 10;
int len = 20;
Agent() {
x = random(width);
y = random(height);
speed = random(-5, 5);
col = cols[(int)random(cols.length)];
}
void display() {
fill(col);
noStroke();
for (int i = 0; i < len; i++) {
update();
}
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
brush(x, y, (int)random(1, 5));
}
void brush(float posx, float posy, int count) {
pushMatrix();
translate(posx, posy);
for (int i = 0; i < count; i++) {
circle(random(5, 10), random(5, 10), random(1, 5));
}
popMatrix();
}
}
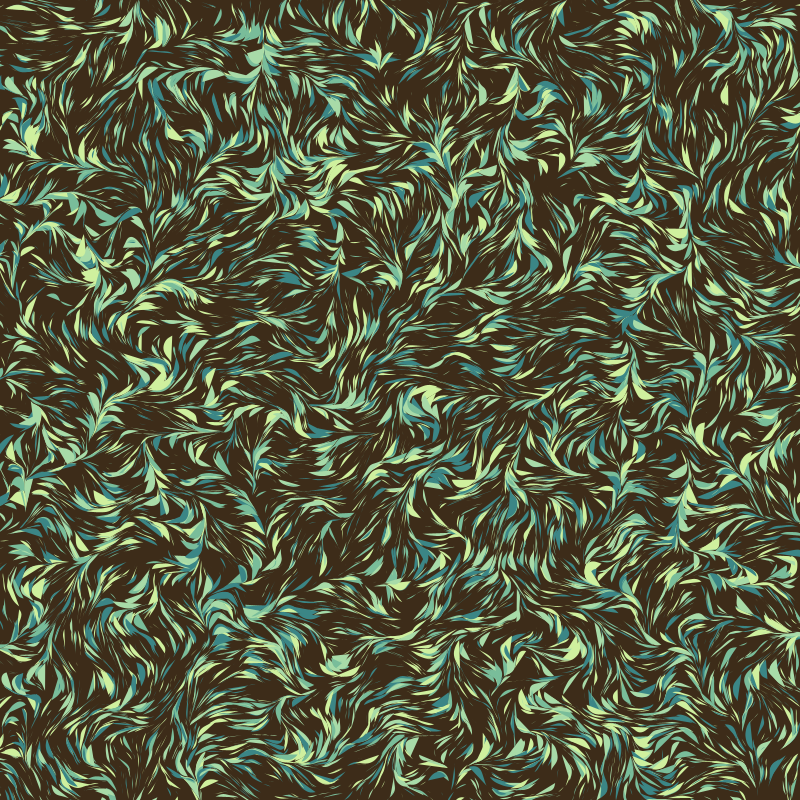
Daily 22.09.08 /// 456 // nat noise / #generativeart #techart #codeart #processing #java
color[] cols = {#cff09e, #79bd9a, #3b8686, #a8dba8};
Agent[] agents;
void setup() {
size(800, 800);
background(#3D2C19);
agents = new Agent[12000];
//noiseSeed(0);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 60, noiseStrength = 10;
int len = 20;
Agent() {
x = random(width);
y = random(height);
speed = random(-2, 2);
col = cols[(int)random(cols.length)];
}
void display() {
fill(col);
noStroke();
beginShape();
for (int i = 0; i < len; i++) {
update();
}
endShape();
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
curveVertex(x, y);
}
}
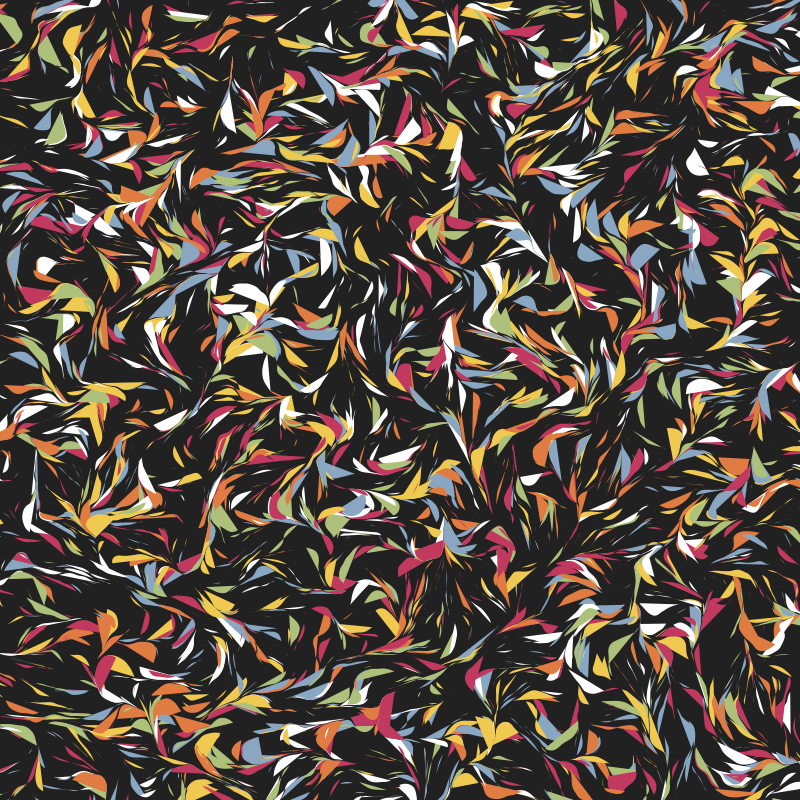
Daily 22.09.07 /// 455 // static noise / #generativeart #techart #codeart #processing #java
color[] cols = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2, #FFFFFF};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[4000];
//noiseSeed(0);
//randomSeed(0);
for (int i = 0; i < agents.length; i++) {
agents[i] = new Agent();
}
noLoop();
}
void draw() {
for (Agent agent : agents) {
agent.display();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed;
color col;
float noiseScale = 60, noiseStrength = 10;
int len = 30;
Agent() {
x = random(width);
y = random(height);
speed = random(-2, 2);
col = cols[(int)random(cols.length)];
}
void display() {
fill(col);
noStroke();
beginShape();
for (int i = 0; i < len; i++) {
update();
}
endShape();
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
curveVertex(x, y);
}
}
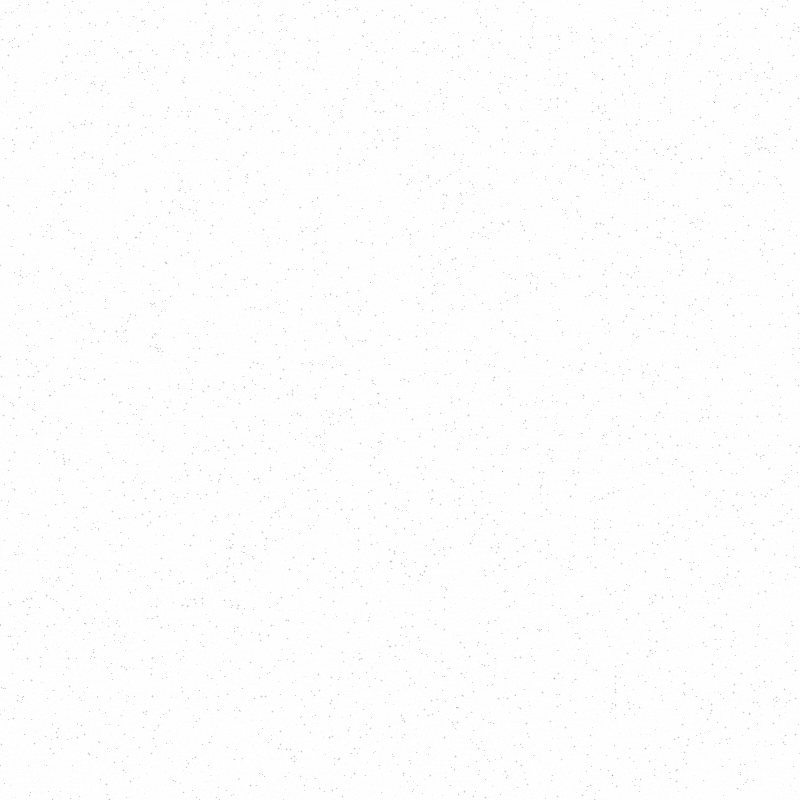
Daily 22.09.06 /// 454 // 3d noise BW / #generativeart #techart #codeart #processing #java
color[] cols = {#000000};
Agent[] agents;
void setup() {
size(800, 800);
background(#FFFFFF);
agents = new Agent[6000];
noiseSeed(2);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y, z;
float speed, size;
color col;
float noiseScale = 100, noiseStrength = 10;
Agent() {
x = random(width);
y = random(height);
size = random(0.2, 2);
speed = random(-2, 2);
z = random(0.2, 0.4);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col, 50);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale, z) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
z += 0.01;
}
}
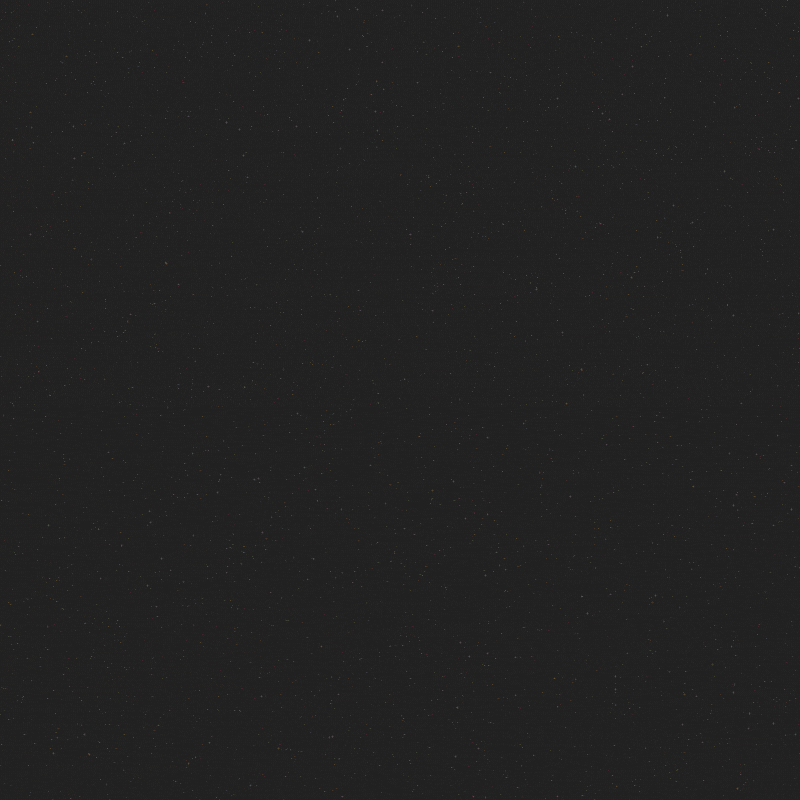
Daily 22.09.05 /// 453 // 3d noise / #generativeart #techart #codeart #processing #java
color[] cols = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2, #FFFFFF};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[6000];
noiseSeed(2);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/line-######.png");
}
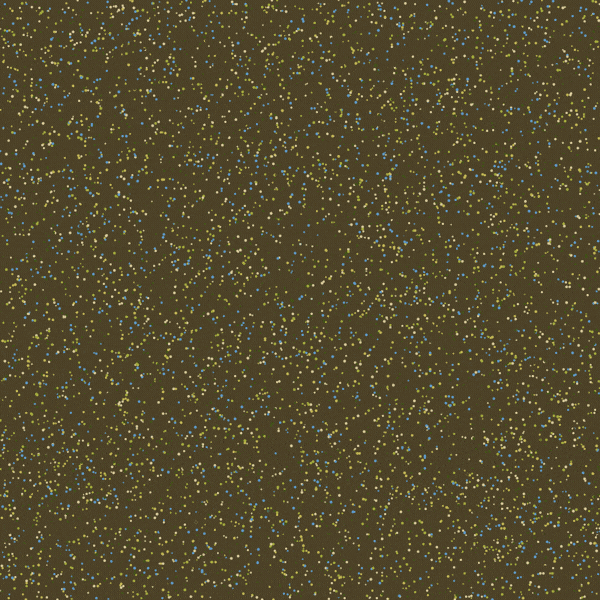
Daily 22.09.02 /// 452 // landscape noise / #generativeart #techart #codeart #processing #java
color[] cols = {#4D4228, #425219, #A1A93D, #BEB153, #D3C598, #5F98C7};
Agent[] agents;
void setup() {
size(800, 800);
background(#4D4228);
agents = new Agent[10000];
noiseSeed(4);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 400, noiseStrength = 8;
Agent() {
x = random(width);
y = random(height);
size = random(2, 4);
speed = random(-2, 2);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
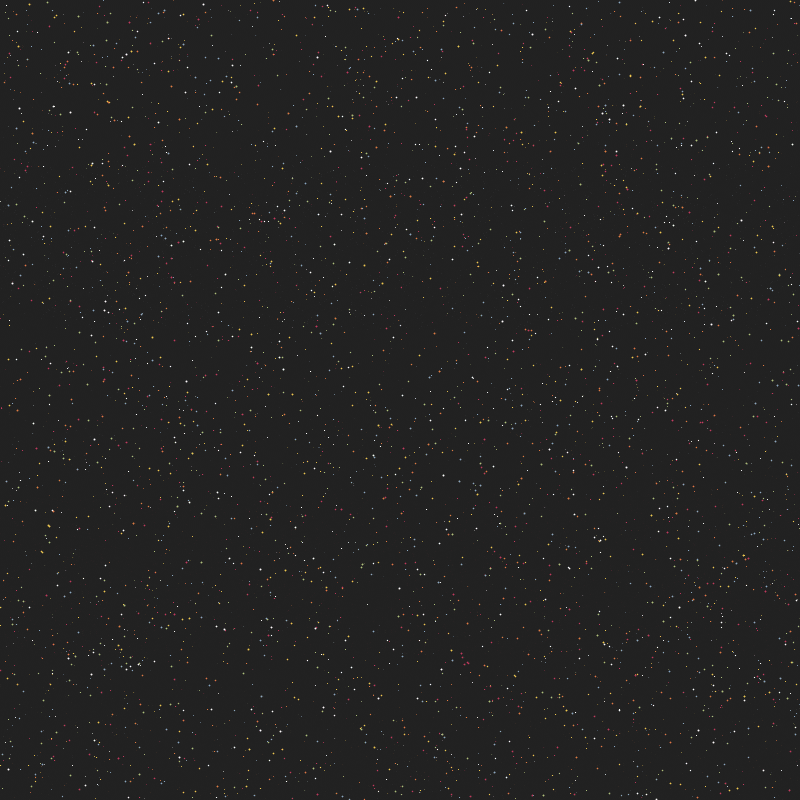
Daily 22.09.01 /// 451 // noiseStrength / #generativeart #techart #codeart #processing #java
color[] cols = {#C1395E, #AEC17B, #F0CA50, #E07B42, #89A7C2, #FFFFFF};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[6000];
noiseSeed(2);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 100, noiseStrength = 10;
Agent() {
x = random(width);
y = random(height);
size = random(0.2, 2);
speed = random(-2, 2);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
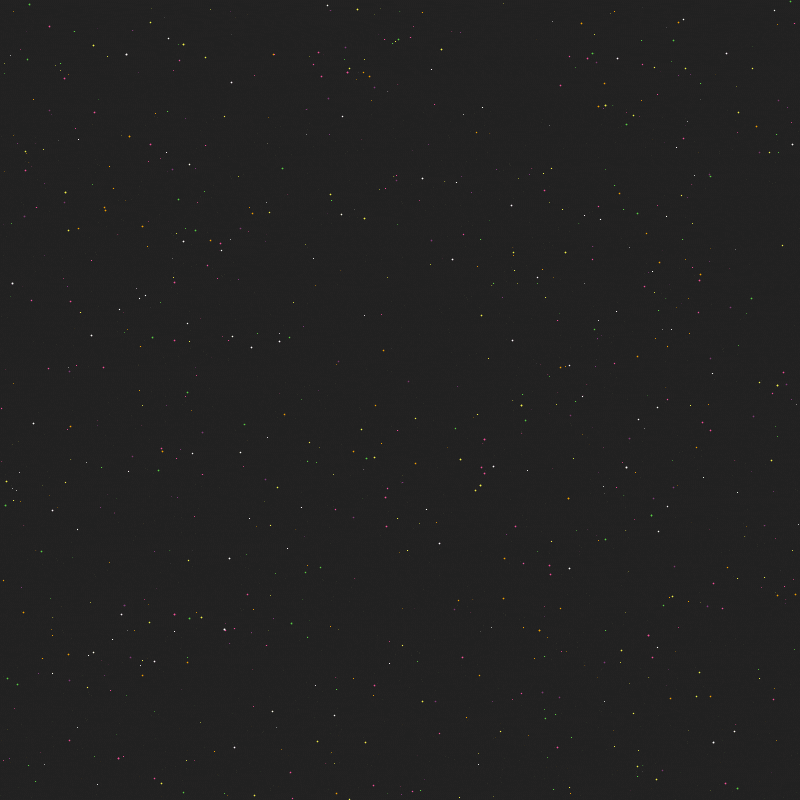
Daily 22.08.30 /// 450 // Multi Agents / #generativeart #techart #codeart #processing #java
color[] cols = {#F24D98, #813B7C, #59D044, #F3A002, #F2F44D, #FFFFFF};
Agent[] agents;
void setup() {
size(800, 800);
background(#212121);
agents = new Agent[1000];
noiseSeed(1);
randomSeed(0);
for(int i = 0; i < agents.length; i++) {
agents[i]= new Agent();
}
}
void draw() {
for (Agent agent: agents) {
agent.display();
agent.update();
}
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 300, noiseStrength = 10;
Agent() {
x = random(width);
y = random(height);
size = random(0.2, 2);
speed = random(-2, 2);
col = cols[(int)random(cols.length)];
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
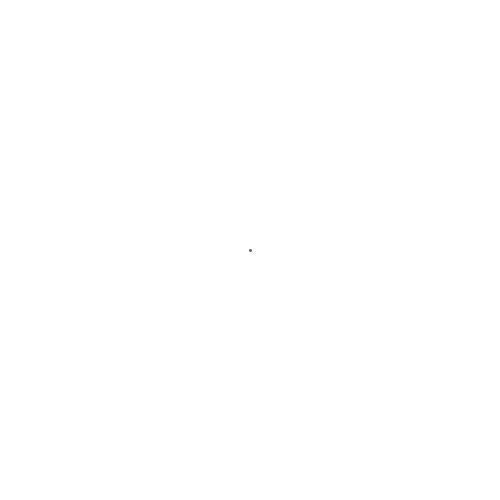
Daily 22.08.29 /// 449 // Noise Agents / #processing #java #generativeart #techart #codeart
Agent agent;
void setup() {
size(500, 500);
background(255);
agent = new Agent();
}
void draw() {
agent.display();
agent.update();
saveFrame("frames/line-######.png");
}
class Agent {
float x, y;
float speed, size;
color col;
float noiseScale = 200, noiseStrength = 300;
Agent() {
x = width/2;
y = height/2;
speed = 1;
size = 3;
col = color(100);
}
void display() {
strokeWeight(size);
stroke(col);
point(x, y);
}
void update() {
float angle = noise(x/noiseScale, y/noiseScale) * noiseStrength;
x += cos(angle) * speed;
y += sin(angle) * speed;
}
}
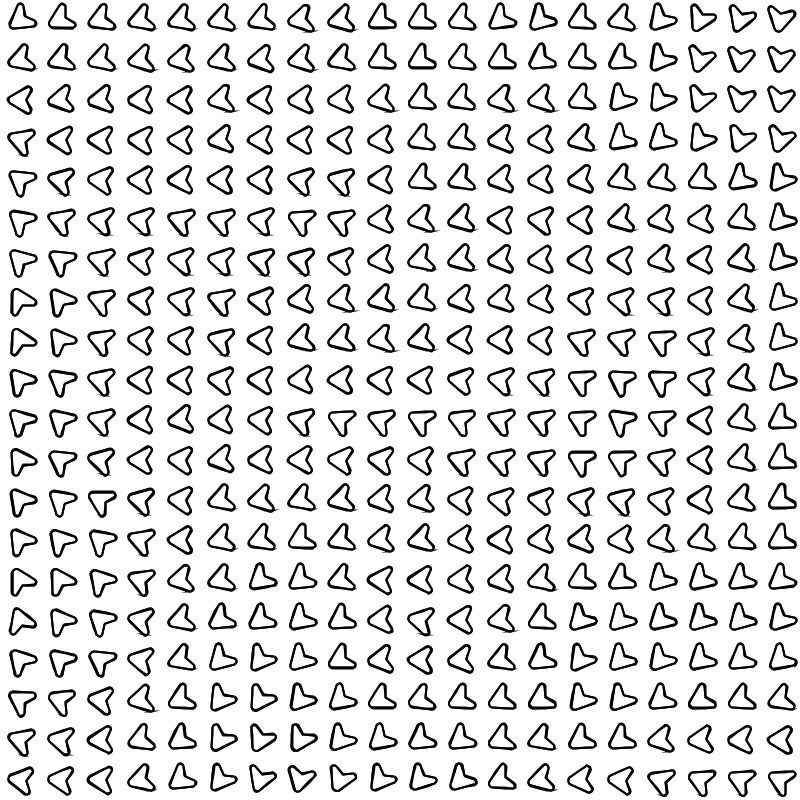
Daily 22.08.26 /// 448 // Flow Field / #processing #java #generativeart #techart #codeart
int cols = 20, rows = 20;
PShape arrow;
FlowField flowfield;
void setup() {
size(800, 800);
background(255);
flowfield = new FlowField(cols, rows);
arrow = loadShape("arrow.svg");
arrow.disableStyle();
strokeWeight(5);
noLoop();
}
void draw() {
translate(20, 20);
flowfield.display();
saveFrame("frames/line-######.png");
}
class FlowField {
PVector[][] field;
int cellW, cellH;
int cols, rows;
float noiseScale = 400;
FlowField(int _cols, int _rows) {
cols = _cols;
rows = _rows;
cellW = width/cols;
cellH = height/rows;
field = new PVector[cols][rows];
init();
}
void init() {
for (int i = 0; i < cols; i++) {
for (int j = 0; j < rows; j++) {
float x = i * cellW;
float y = j * cellH;
float angle = map(noise(x/noiseScale, y/noiseScale), 0, 1, 0, TWO_PI);
PVector v = new PVector(cos(angle), sin(angle));
field[i][j] = v;
}
}
}
void display() {
for (int i = 0; i < cols; i++) {
for (int j = 0; j < rows; j++) {
pushMatrix();
translate(i * cellW, j * cellH);
rotate(field[i][j].heading());
scale(0.4);
shape(arrow, -arrow.width/2, -arrow.height/2);
popMatrix();
}
}
}
}
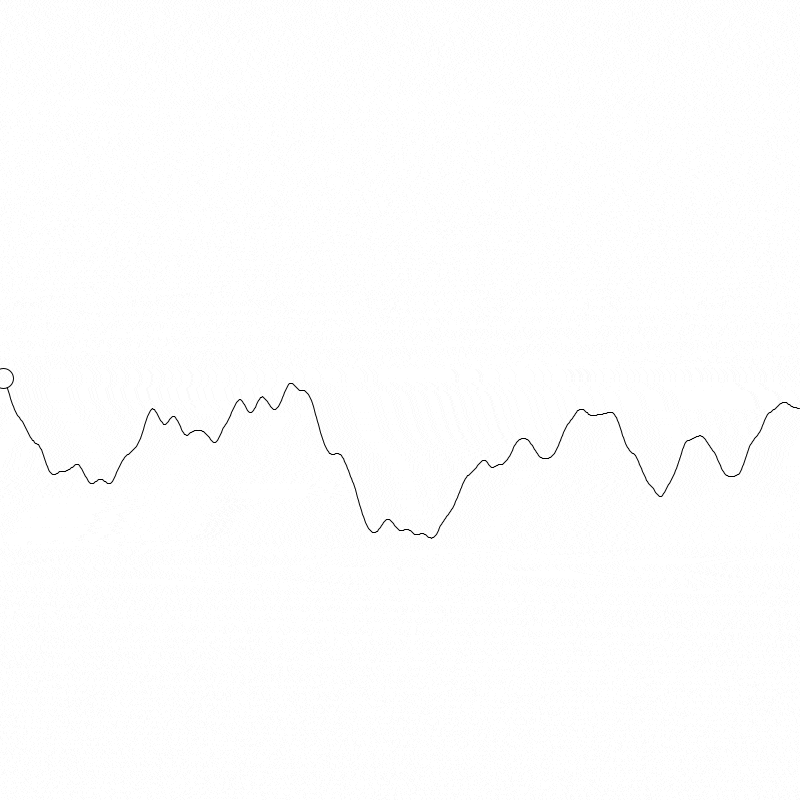
Daily 22.08.24 /// 447 // Noise / #processing #java #generativeart #techart #codeart
float x, y;
float scale = 100;
float strength = 400;
PShape wave;
void setup() {
size(800, 800);
background(255);
y = height/3;
wave = createShape();
wave.beginShape();
for (float x = 0; x < width; x++) {
y = noise(x/scale) * strength;
wave.vertex(x, y + height/3);
}
wave.endShape();
}
void draw() {
background (255);
shape(wave);
circle(x, y + height/3, 20);
x++;
y = noise(x/scale) * strength;
if (x > width) x = 0;
saveFrame("frames/line-######.png");
}