Dailies: Taking an hour each day to prototype and learn new techniques.
Learning some basics from a tutorial from Unity
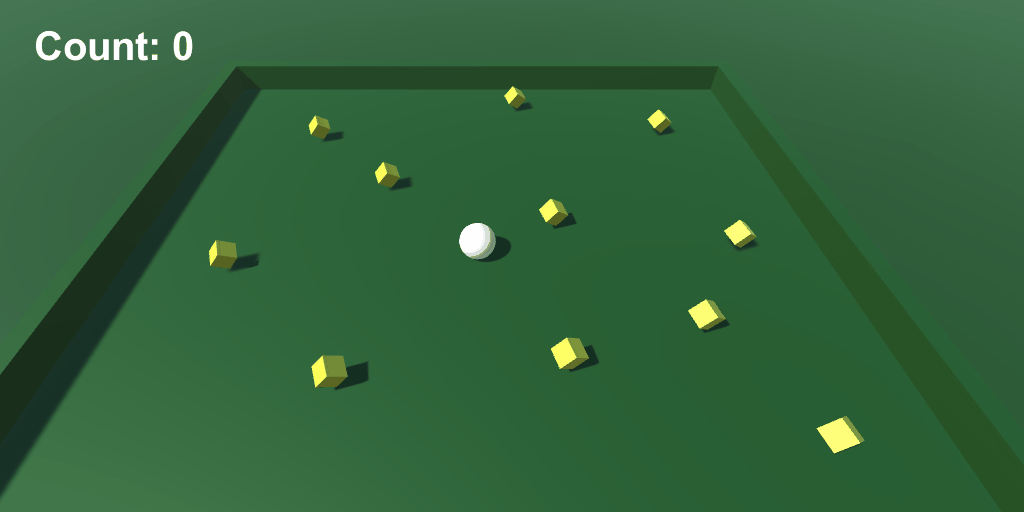
Learning some basic code to count colliders and send a message when complete. Pretty basic standard stuff but now I know.
Daily 19.01.29 /// 189 // roll a ball 2 / #madewithunity #gamedev
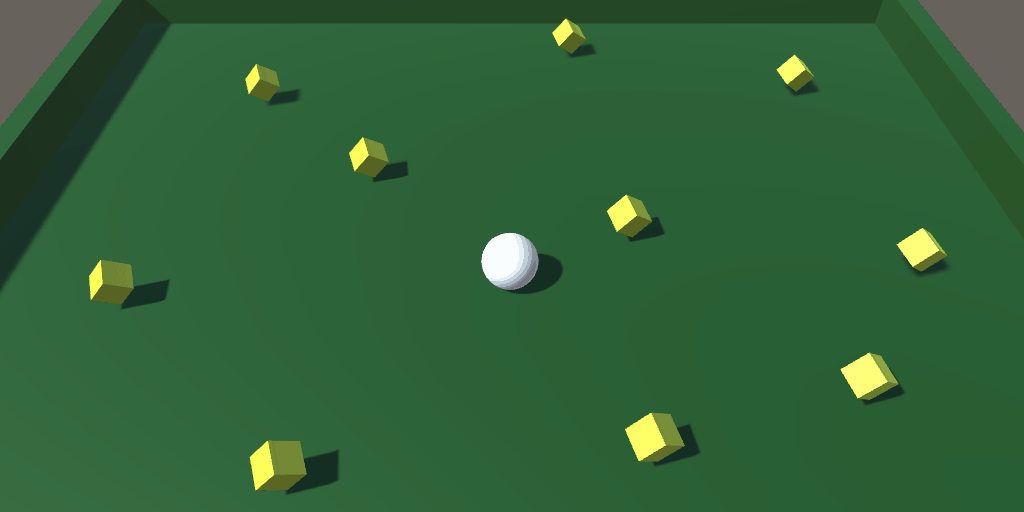
Usually use @unity3d for VFX but super inspired by #GGJ19 and plan to do some game jams this year for shits and giggles. Plowing through some @unity3d tuts to learn the fundamentals.
Daily 19.01.28 /// 188 // roll a ball 1 / #madewithunity #gamedev
PlayerController
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerController : MonoBehaviour {
public float speed;
public Text countText;
public Text winText;
private Rigidbody rb;
private int count;
// Use this for initialization
void Start () {
rb = GetComponent<Rigidbody>();
count = 0;
SetCountText();
winText.text = "";
}
// Update is called once per frame
void Update () {
}
void FixedUpdate()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
rb.AddForce(movement * speed);
}
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Pickup"))
{
other.gameObject.SetActive(false);
count = count + 1;
SetCountText();
}
}
void SetCountText ()
{
countText.text = "Count: " + count.ToString ();
if (count >= 12)
{
winText.text = "You Win!";
}
}
}
CameraController
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraController : MonoBehaviour {
public GameObject player;
private Vector3 offset;
// Use this for initialization
void Start () {
offset = transform.position - player.transform.position;
}
// Update is called once per frame
void LateUpdate () {
transform.position = player.transform.position + offset;
}
}
Rotator
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Rotator : MonoBehaviour {
// Update is called once per frame
void Update () {
transform.Rotate(new Vector3(15, 30, 45) * Time.deltaTime);
}
}